Python Getting Started: A Beginner’s Guide to Your First Coding Steps
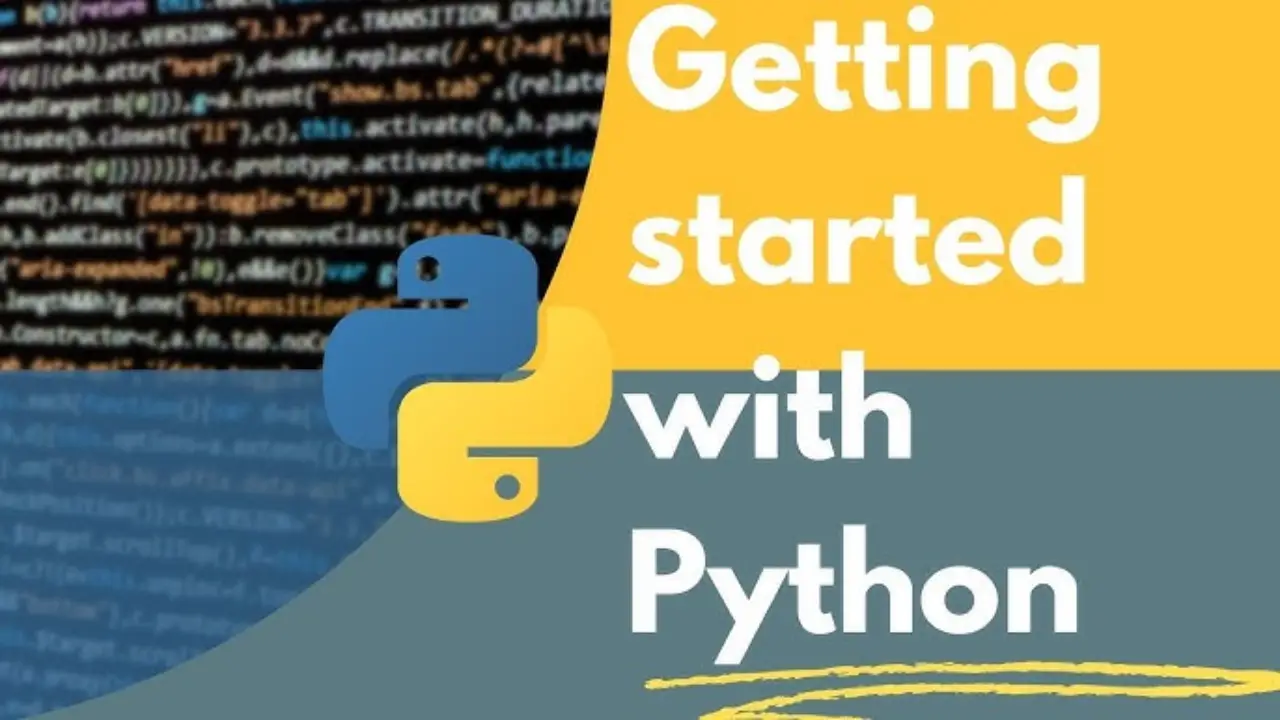
Python Getting Started: A Beginner’s Guide to Your First Coding Steps
Python is one of the most popular programming languages today, praised for its simplicity, readability, and versatility. Whether you're looking to learn programming for the first time or switch from another language, Python offers a clear path forward. This comprehensive guide will take you step by step through the process of getting started with Python, from installation to writing your first Python programs, and setting up your environment. By the end, you'll have the knowledge you need to embark on your coding journey with confidence.
1. What Is Python?
Before you start coding, it's essential to understand what Python is and why it's so widely used. Python is a high-level, interpreted programming language designed to be easy to read and write. Its syntax closely resembles the English language, making it beginner-friendly. Since its creation by Guido van Rossum in 1991, Python has become one of the top programming languages for web development, data analysis, artificial intelligence, and more.
Python’s key strengths include:
- Simplicity: Python’s syntax is easy to learn and reduces the complexity of coding tasks.
- Versatility: Python can be used for web development, machine learning, data science, automation, and much more.
- Large Ecosystem: Python has a vast collection of libraries and frameworks, allowing you to extend its functionality for various applications.
2. Why Choose Python?
There are many reasons why beginners and experienced developers alike gravitate toward Python. Here’s why you should choose Python as your starting point for learning programming:
- Easy to Learn: Python’s readability and straightforward syntax make it one of the best languages for beginners.
- Widely Used: Python is used in various fields, from web development (Django, Flask) to data science (Pandas, NumPy) and artificial intelligence (TensorFlow, Keras).
- Great Community Support: Python has a vast, active community of developers who contribute to forums, tutorials, and open-source libraries, making it easy for beginners to find support.
- Cross-Platform: Python runs on all major operating systems, including Windows, macOS, and Linux, which ensures that you can develop applications across multiple platforms.
3. Setting Up Your Python Development Environment
Before diving into coding, you need to set up your Python development environment. This includes installing Python and choosing an appropriate Integrated Development Environment (IDE) or text editor.
Installing Python
Download Python: Visit the official Python website at python.org. Choose the latest version of Python 3.x (as Python 2 is no longer supported).
Run the Installer: Once the installer is downloaded, open it and follow the instructions. On Windows, make sure to check the box that says “Add Python to PATH” before continuing with the installation process.
Verify Installation: After installation is complete, open the command prompt (Windows) or terminal (macOS/Linux), and type the following command:
python --version
This should return the installed version of Python.
Choosing a Code Editor or IDE
While you can technically write Python code using any text editor, a specialized code editor or IDE can make your life much easier. Some popular choices for Python development include:
- IDLE: Python comes with its own basic IDE called IDLE, which is simple and easy to use for beginners.
- Visual Studio Code (VS Code): A free, powerful code editor with support for Python through extensions.
- PyCharm: A full-featured Python IDE, perfect for larger projects and professional development.
- Jupyter Notebook: If you're planning to work with data science or machine learning, Jupyter Notebooks are great for writing and running Python code in an interactive, cell-based format.
4. Writing Your First Python Program
Let’s dive into writing some code! The first program every programmer writes is typically called the "Hello, World!" program. Here’s how to do it in Python:
Create a Python File: Open your text editor or IDE, and create a new file. Name it hello.py
.
Write Your Code: In the new file, write the following Python code:
print("Hello, World!")
Run Your Program: Save the file and run it using your terminal or IDE. If you're using the terminal, navigate to the folder where your file is located and type:
python hello.py
Output: You should see Hello, World!
printed on your screen.
5. Understanding Python Basics
Now that you’ve written your first program, let’s dive into the fundamental concepts of Python.
Variables and Data Types
In Python, variables are used to store data. The data stored in variables can be of various types:
- Strings: Text data, e.g.,
"Hello, Python!"
- Integers: Whole numbers, e.g.,
42
- Floats: Decimal numbers, e.g.,
3.14
- Booleans: True or False values, e.g.,
True
- Lists: Ordered collections of items, e.g.,
[1, 2, 3]
- Dictionaries: Key-value pairs, e.g.,
{"name": "Alice", "age": 30}
Here’s an example of defining variables in Python:
name = "Alice"
age = 30
height = 5.6
is_student = False
Operators
Operators in Python allow you to perform operations on variables. Some common types of operators include:
- Arithmetic Operators:
+
,-
,*
,/
,%
(addition, subtraction, multiplication, division, modulus) - Comparison Operators:
==
,!=
,>
,<
,>=
,<=
(to compare values) - Logical Operators:
and
,or
,not
(for logical operations) - Assignment Operators:
=
,+=
,-=
(for assigning or modifying values)
Example:
x = 5
y = 3
sum = x + y
print(sum) # Output: 8
6. Control Flow: Conditional Statements and Loops
One of the most essential aspects of programming is controlling the flow of your program. Python provides various ways to control the flow, including conditional statements and loops.
If Statements
if
statements allow you to run different code based on certain conditions.
age = 20
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
Loops
Loops allow you to repeat a block of code multiple times. Python has two main types of loops:
For Loop: Typically used to iterate over a sequence (like a list or range).
for i in range(5):
print(i) # Output: 0 1 2 3 4
While Loop: Executes a block of code as long as a condition is true.
count = 0
while count < 5:
print(count) # Output: 0 1 2 3 4
count += 1
7. Functions: Organizing Your Code
Functions allow you to organize your code into reusable blocks. Functions are defined using the def
keyword in Python.
def greet(name):
print(f"Hello, {name}!")
greet("Alice") # Output: Hello, Alice!
Functions can accept arguments and return values, making them flexible and powerful for organizing complex tasks.
8. Libraries and Frameworks in Python
One of Python's key strengths is its extensive library ecosystem. Libraries provide pre-written code for specific tasks, saving you time and effort. Here are some popular libraries you might encounter:
- NumPy: For numerical computing and working with large datasets.
- Pandas: For data manipulation and analysis.
- Matplotlib: For creating visualizations and plots.
- Flask: A lightweight web framework for building web applications.
- Django: A high-level web framework for rapid development of secure and maintainable websites.
To install a Python library, you use the Python package manager pip
:
pip install numpy
9. Debugging and Best Practices
As you write Python code, you'll inevitably encounter bugs. Debugging is the process of finding and fixing these issues. Here are some best practices for debugging and writing clean Python code:
- Read Error Messages Carefully: Python provides detailed error messages that can help you identify where something went wrong.
- Use Print Statements: Print values to the console to check variables and the flow of your program.
- Adhere to PEP 8: Python's style guide provides recommendations for writing clean, readable code, such as using consistent indentation and meaningful variable names.
- Version Control: Use Git to track changes in your code and collaborate with others.
10. Next Steps After Mastering the Basics
Once you’ve mastered the basics of Python, it’s time to take your skills to the next level. Here are some ways you can continue your learning journey:
- Learn Object-Oriented Programming (OOP): OOP is a programming paradigm that organizes your code into classes and objects. Python fully supports OOP.
- Build Projects: Apply what you’ve learned by building small projects. This could be anything from a simple calculator to a to-do list app or a personal website.
- Contribute to Open Source: GitHub is home to many open-source Python projects. Contributing to these projects can be a great way to learn from others and enhance your coding skills.
- Explore Advanced Topics: Learn about advanced Python concepts like decorators, generators, and multi-threading.
Conclusion
Getting started with Python is an exciting and rewarding journey. With its beginner-friendly syntax, versatility, and vast library ecosystem, Python is the perfect language to kickstart your programming career. By following the steps outlined in this guide, you can set up your environment, learn the basics, and begin writing Python code that will serve as the foundation for your future programming endeavors. Keep practicing, stay curious, and enjoy your coding journey!
Happy coding!
0 Comments
No Comment Available