Beginner's Step-by-Step Guide to Creating a Discord Bot Using Node.js
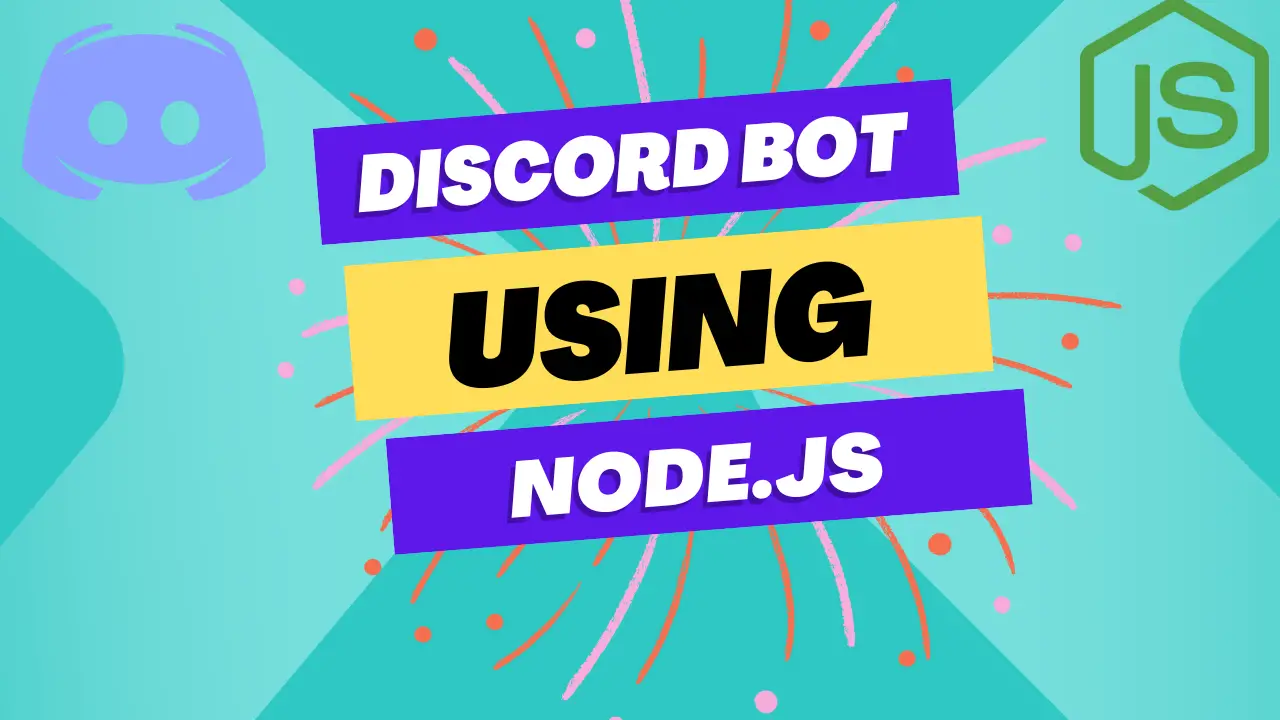
Beginner's Step-by-Step Guide to Creating a Discord Bot Using Node.js
Creating a Discord bot can be an exciting and rewarding project for developers, and Node.js offers an excellent platform to get started. This detailed guide will take you through the entire process of building a Discord bot from scratch. You’ll learn how to set up your development environment, write the code for your bot, and deploy it to a Discord server. By the end of this tutorial, you’ll have a fully operational Discord bot equipped with custom features to enhance your server.
Prerequisites
Before we dive into the code, let’s go over the basic prerequisites:
- Node.js: You need to have Node.js installed. If you haven’t installed it yet, go to the official Node.js website and download the LTS version.
- Discord Account: You need a Discord account to create and manage your bot.
- Code Editor: Use a code editor like Visual Studio Code for writing your bot’s code.
- Command Line Interface (CLI): Basic knowledge of using the terminal or command prompt is essential for managing packages and running the bot.
Now that you're all set, let’s begin!
Step 1: Set Up Your Discord Bot Application
1.1. Create a New Application in Discord
- Go to the Discord Developer Portal and log in with your Discord account.
- Click on the “New Application” button.
- Give your application a name, and then click "Create."
1.2. Set Up Your Bot
- Select the application you just created and go to the Bot section on the left-hand sidebar.
- Click the Add Bot button, then confirm by clicking "Yes, do it!".
- Once the bot is created, you will see the TOKEN. This token is required to authenticate your bot with Discord’s API. Make sure to keep it safe, as anyone with this token can control your bot.
Step 2: Set Up Your Development Environment
2.1. Initialize a Node.js Project
Open your terminal or command prompt.
Navigate to the directory where you want to create your bot project.
Run the following command to initialize a new Node.js project:
npm init -y
This will create a package.json
file in your directory, which is used to manage your project’s dependencies.
2.2. Install Discord.js
We will be using the discord.js
library to interact with Discord’s API. Install it by running the following command:
npm install discord.js
This will download and install the discord.js
package and its dependencies.
2.3. Install Additional Packages (Optional)
You might also want to install some additional packages, such as dotenv
for managing environment variables (like your bot token). To install dotenv
, run:
npm install dotenv
Now, let’s move on to the actual coding part!
Step 3: Write Your First Discord Bot Code
3.1. Create a New JavaScript File
In your project directory, create a new file named bot.js
(or any name you prefer). This will be the file where we write the bot’s logic.
3.2. Set Up the Basic Bot Structure
Start by requiring the necessary libraries (discord.js
and dotenv
if you’re using it):
const { Client, GatewayIntentBits } = require('discord.js');
require('dotenv').config(); // For managing environment variables
Now, create a new instance of the Client
class. This object will represent your bot:
const client = new Client({
intents: [
GatewayIntentBits.Guilds,
GatewayIntentBits.GuildMessages,
GatewayIntentBits.MessageContent,
],
});
3.3. Log In Using Your Bot’s Token
Add the following code to authenticate your bot using the bot token you obtained from the Discord Developer Portal:
client.login(process.env.BOT_TOKEN);
Make sure to add the bot token to your .env
file if you're using dotenv
to manage your environment variables. Your .env
file should look something like this:
BOT_TOKEN=YOUR_BOT_TOKEN_HERE
3.4. Set Up Event Listeners
Next, let’s add some event listeners to handle basic interactions with the bot. The most common event is the messageCreate
event, which listens for messages sent in the server.
client.on('messageCreate', (message) => {
if (message.author.bot) return; // Prevents the bot from responding to itself
if (message.content.toLowerCase() === 'ping') {
message.reply('Pong!');
}
});
In this example, the bot will reply with "Pong!" whenever someone types "ping" in the chat.
Step 4: Run Your Bot
4.1. Run the Bot Locally
Now that the bot is set up, it's time to run it! Open the terminal and execute the following command in your project directory:
node bot.js
If everything is set up correctly, your bot should now be online! You can go to your Discord server and test it by typing "ping." The bot should reply with "Pong!"
4.2. Invite Your Bot to a Discord Server
To invite your bot to a server, go back to the Discord Developer Portal:
- Go to the OAuth2 section of your bot’s application page.
- Under OAuth2 URL Generator, select the bot scope and the administrator permissions (or select specific permissions based on your bot’s functionality).
- Copy the generated URL and open it in your browser.
- Select a server and click "Authorize."
Your bot will now be added to your server, and you can start interacting with it!
Step 5: Enhance Your Bot with Additional Features
Now that you have a basic bot up and running, let's add some additional features to make it more functional.
5.1. Command Prefix
It’s a good idea to use a command prefix to distinguish bot commands from regular messages. For example, we can change the code to respond to commands that begin with !
:
client.on('messageCreate', (message) => {
if (message.author.bot) return;
if (message.content.startsWith('!ping')) {
message.reply('Pong!');
}
});
5.2. More Commands
Let’s add a couple of more commands to show how easy it is to extend your bot’s functionality:
client.on('messageCreate', (message) => {
if (message.author.bot) return;
const args = message.content.slice(1).trim().split(/ +/); // Split command arguments
const command = args.shift().toLowerCase();
if (command === 'ping') {
message.reply('Pong!');
} else if (command === 'hello') {
message.reply('Hello, World!');
} else if (command === 'userinfo') {
const user = message.author;
message.reply(`Your username is ${user.username} and your ID is ${user.id}`);
}
});
Now, your bot can respond to !ping
, !hello
, and !userinfo
commands.
5.3. Adding More Complex Features
You can add features such as music commands, moderation tools, and more. For example, if you wanted your bot to play music from YouTube, you could use libraries like discord.js-music.
Step 6: Deploy Your Bot
Once you’ve built your bot and tested it locally, you may want to deploy it so it can run 24/7. Popular options include:
- Heroku: A free cloud platform where you can easily deploy Node.js apps.
- Replit: An online IDE that allows you to run your bot in the cloud.
- AWS, DigitalOcean, or Google Cloud: These services offer virtual machines that you can use to run your bot on a cloud server.
Each of these platforms has documentation on how to deploy Node.js apps, so follow the steps on their respective sites.
Conclusion
Building a Discord bot with Node.js is an excellent project for beginners, offering a great way to learn both Node.js and how to interact with web APIs. In this guide, you learned how to set up a basic bot, write simple commands, and deploy your bot. As you become more comfortable, you can extend your bot with advanced features like music playback, games, and more. Happy coding, and enjoy building your very own Discord bot!
This tutorial provides a simple yet thorough guide for beginners to create their own Discord bot using Node.js. If you'd like to continue developing your bot further, consider exploring the official discord.js documentation.
0 Comments
No Comment Available