How to Set Up a React.js Server for Scalable Web Applications
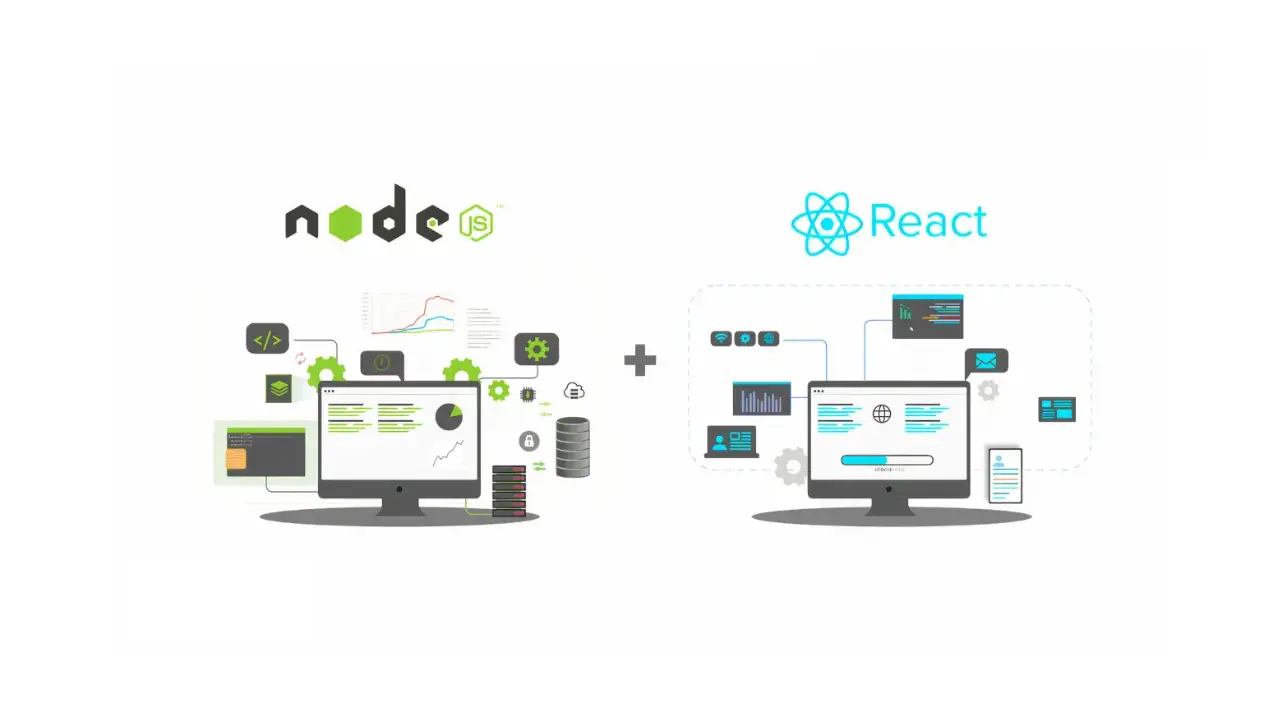
How to Set Up a React.js Server for Scalable Web Applications
React.js, a powerful JavaScript library for building user interfaces, is known for its efficiency and flexibility. While React is typically used for building the front-end of web applications, combining it with a server setup can improve performance, SEO, and user experience. By leveraging server-side rendering (SSR) or static site generation (SSG), developers can create React apps that are faster, more scalable, and production-ready.
In this guide, we’ll walk you through the entire process of setting up a React.js server for scalable web applications. Whether you're new to React or experienced with front-end development, this post will provide the knowledge you need to build high-performance React apps and deploy them to production.
Table of Contents
Why React.js Server Setup Matters
- The Benefits of Server-Side Rendering (SSR)
- Scalability Challenges in Web Applications
- How React.js Server Enhances Performance
Prerequisites for Setting Up a React.js Server
- What You Need Before You Begin
- Understanding React.js Basics
- Tools and Libraries You’ll Need
Setting Up the Development Environment
- Installing Node.js and npm
- Installing React and Creating Your First React App
- Configuring Your Development Server
Understanding Server-Side Rendering (SSR)
- What is SSR and Why Does it Matter?
- The Benefits of SSR in React.js
- How SSR Improves SEO and Performance
Setting Up SSR with React.js
- Creating a Basic SSR React App
- Configuring React Router for SSR
- Integrating with Express.js for SSR
- Implementing React.js Hydration for Client-Side Interactivity
Optimizing React.js Server for Scalability
- Server Optimization Techniques
- Caching Strategies for SSR Apps
- Load Balancing for High Traffic Applications
- Using CDNs for Static Assets
Deploying Your React.js Server to Production
- Preparing Your Application for Deployment
- Hosting React.js Apps with Popular Platforms (AWS, Heroku, DigitalOcean)
- Continuous Integration/Continuous Deployment (CI/CD) for React.js
- Handling Environment Variables for Production
Best Practices for Building Scalable React Applications
- Code Splitting and Lazy Loading
- Optimizing React Rendering with Memoization
- Server-Side Caching and Optimizing Database Queries
- Managing State Effectively in Large Applications
Testing and Debugging Your React.js Server
- Unit and Integration Testing with Jest and React Testing Library
- Debugging React SSR Issues
- Performance Monitoring with Tools like Lighthouse
Troubleshooting and Common Challenges
- Handling SEO with SSR and SSG
- Debugging Common React SSR Errors
- Solutions for Performance Bottlenecks
Conclusion
- Recap of Key Concepts
- Why Server-Side Rendering is Essential for Scalability
- Resources for Further Learning
1. Why React.js Server Setup Matters
The Benefits of Server-Side Rendering (SSR)
Server-Side Rendering (SSR) is the process of rendering a web page on the server instead of in the browser. By generating the initial HTML on the server and sending it to the client, SSR significantly improves performance and SEO. When users request a page, they receive a fully-rendered HTML page instead of waiting for JavaScript to load and render the content.
- Faster Initial Load: Since the server renders the content, users receive the content faster.
- Improved SEO: Search engines can crawl fully-rendered HTML, improving the visibility of your app.
- Enhanced User Experience: SSR helps reduce the time-to-interactive for the page.
Scalability Challenges in Web Applications
As your React.js application grows, scalability becomes a key concern. A well-architected server setup ensures that your app can handle increasing traffic without compromising performance. Challenges include handling high traffic loads, optimizing resource usage, and ensuring fast response times.
How React.js Server Enhances Performance
With React.js, leveraging server-side rendering and other optimizations allows developers to build highly performant applications that scale easily. By rendering components on the server, reducing client-side rendering time, and implementing caching mechanisms, you can create applications that respond quickly even as they grow in complexity.
2. Prerequisites for Setting Up a React.js Server
Before diving into setting up a React.js server, there are a few things you’ll need:
What You Need Before You Begin
- Node.js: React.js runs on Node.js, so you’ll need to have it installed on your machine.
- npm or Yarn: These package managers are used to install the necessary dependencies for React.js.
- Code Editor: A text editor such as Visual Studio Code will help you write and manage your React.js code.
- Basic Knowledge of React: Familiarity with React components, state, props, and hooks is essential.
Understanding React.js Basics
Before proceeding with server setup, ensure you understand React basics such as JSX, functional components, state management, and props. These foundational concepts will make it easier to build React apps and integrate them with a server.
Tools and Libraries You’ll Need
- React: The core library for building the UI.
- React Router: Used for routing in React applications.
- Express.js: A minimalist web server framework for handling HTTP requests in the Node.js environment.
- Babel: JavaScript compiler used for compiling JSX and ES6 code into browser-compatible JavaScript.
3. Setting Up the Development Environment
Installing Node.js and npm
To start, install Node.js from the official website. npm (Node Package Manager) comes bundled with Node.js and will be used to manage your project dependencies.
Installing React and Creating Your First React App
Once Node.js is installed, you can create your React app using Create React App (CRA). This tool sets up the initial environment with all the necessary configurations.
npx create-react-app my-app
cd my-app
npm start
This command will set up a development environment and start your React app locally.
Configuring Your Development Server
If you plan to use server-side rendering, you'll need to set up an Express.js server. Install Express.js in your project:
npm install express
Then create a basic server setup in server.js
to serve your React app.
4. Understanding Server-Side Rendering (SSR)
What is SSR and Why Does it Matter?
Server-Side Rendering (SSR) refers to the process of rendering a React app on the server and sending the HTML to the browser, as opposed to client-side rendering (CSR), where the React app is rendered in the browser. SSR can significantly improve the perceived performance and SEO of your app.
The Benefits of SSR in React.js
SSR with React can:
- Improve SEO: Search engines can crawl fully-rendered HTML, improving search rankings.
- Speed up initial load: The server sends pre-rendered HTML, leading to faster load times.
- Provide better UX: Users see content faster because the server handles rendering.
How SSR Improves SEO and Performance
With SSR, the server pre-renders the content and sends it as a complete HTML page. This allows search engines to index your content and improves load times. By rendering on the server, you reduce the amount of JavaScript the browser has to execute on initial load.
5. Setting Up SSR with React.js
Creating a Basic SSR React App
Set up a basic React app and implement SSR with Express.js. You'll need to modify the React app’s entry point and use ReactDOMServer.renderToString()
to render the React components into HTML on the server.
Example of SSR in React:
import React from 'react';
import ReactDOMServer from 'react-dom/server';
import App from './App';
const server = express();
server.use('*', (req, res) => {
const content = ReactDOMServer.renderToString(<App />);
res.send(content);
});
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
Configuring React Router for SSR
To handle routing in an SSR app, integrate react-router-dom
with Express. Use the router to determine which components to render on the server based on the requested URL.
6. Optimizing React.js Server for Scalability
Server Optimization Techniques
- Caching: Cache frequently requested pages to reduce server load.
- Load Balancing: Use multiple servers to distribute traffic evenly.
- Database Optimization: Optimize database queries to prevent bottlenecks.
Using CDNs for Static Assets
A Content Delivery Network (CDN) can serve static assets like images, stylesheets, and scripts, reducing server load and improving response times for users globally.
7. Deploying Your React.js Server to Production
Preparing Your Application for Deployment
Before deploying, ensure that your React app is production-ready. Use Webpack to bundle your assets, optimize images, and minimize JavaScript.
Hosting React.js Apps with Popular Platforms
Deploy your app to platforms like AWS, Heroku, or DigitalOcean. Each platform offers tools for deploying Node.js apps with minimal configuration.
Conclusion
Setting up a React.js server for scalable web applications is crucial for building fast, efficient, and SEO-friendly apps. By implementing server-side rendering, optimizing your server, and following best practices, you can create React apps that handle high traffic and provide great user experiences.
0 Comments
No Comment Available