How Well Do You Know React? Take This Ultimate React Quiz
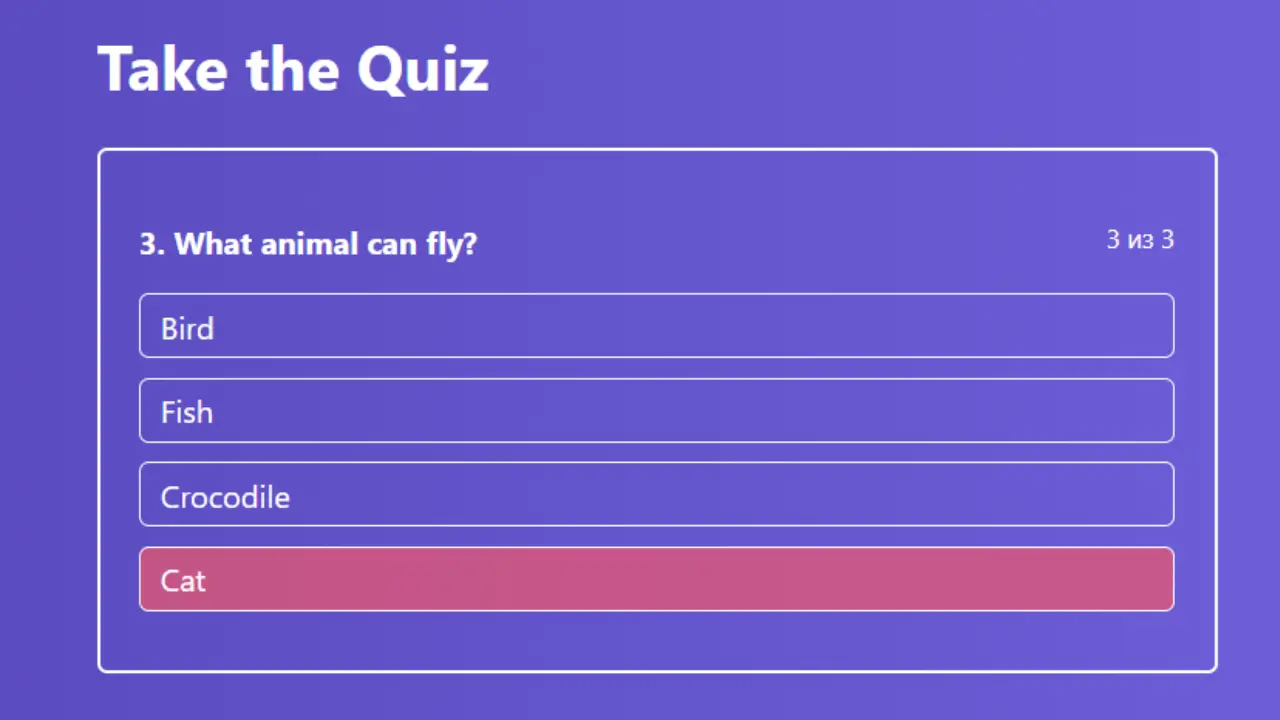
How Well Do You Know React? Take This Ultimate React Quiz
Introduction
React is one of the most widely used JavaScript libraries for building user interfaces. Whether you’re a beginner or an experienced developer, it’s essential to gauge your understanding of React concepts, components, and best practices regularly. One great way to measure your knowledge is by taking a React quiz. But what exactly should you know about React to be considered proficient?
In this article, we’ll provide you with a comprehensive quiz designed to test your understanding of React fundamentals, advanced concepts, and best practices. We’ll guide you through each section, explain key concepts, and offer insights into why these topics are essential for React developers.
The Importance of Testing Your React Knowledge
Why React Knowledge Matters
React has become the go-to library for building dynamic, user-friendly interfaces. The library’s declarative nature and component-based architecture have made it a game-changer in the world of front-end development. Understanding React thoroughly allows developers to create faster, scalable, and more maintainable applications.
By keeping your React knowledge sharp, you’ll be able to develop high-quality web apps, debug issues effectively, and stay ahead of new features and best practices.
How Quizzes Can Enhance Your Learning
Taking quizzes is an excellent way to assess your strengths and areas for improvement. Quizzes help reinforce concepts, highlight gaps in your knowledge, and keep you motivated to continue learning. React quizzes can test both fundamental topics like JSX and state management, as well as advanced subjects like hooks, performance optimization, and testing. Whether you score high or low, each question will give you valuable insights that can help refine your React skills.
React’s Impact on Web Development
React has transformed how developers approach building UIs. Thanks to its component-driven architecture and virtual DOM, React allows developers to build high-performance web applications more efficiently. With its extensive ecosystem and community support, React also integrates seamlessly with other libraries and tools, making it a versatile choice for developers worldwide.
React Basics: What Every Developer Should Know
React Components
React components are the fundamental building blocks of a React application. Components allow you to break down the UI into smaller, reusable pieces, making it easier to manage, test, and update your app. Components can be class-based or functional, with functional components being the recommended approach due to the introduction of hooks.
- Functional Components: These are JavaScript functions that return JSX, representing the UI of the component.
- Class Components: These were traditionally used to manage state and lifecycle methods, but they’re now less common in favor of hooks.
Example of a simple functional component:
function Welcome(props) {
return <h1>Hello, {props.name}!</h1>;
}
JSX Syntax
JSX (JavaScript XML) allows you to write HTML-like syntax inside JavaScript code. It’s a key feature of React that makes the code more readable and expressive. JSX gets transpiled to regular JavaScript, and React uses this syntax to efficiently update the DOM.
Here’s an example of JSX in action:
const element = <h1>Hello, world!</h1>;
Props and State
Props and state are essential concepts in React.
- Props: These are used to pass data from parent components to child components. They are read-only and can’t be modified by the receiving component.
- State: State refers to the internal data of a component that can change over time. Unlike props, state is mutable, and React re-renders the component when state changes.
Example of a component using both props and state:
import React, { useState } from 'react';
function Counter(props) {
const [count, setCount] = useState(0);
return (
<div>
<p>{props.message}</p>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Handling Events in React
Handling events in React is similar to regular JavaScript, but there are a few differences. For example, React uses camelCase for event names (e.g., onClick
instead of onclick
). You also need to pass an event handler function that is bound to the component.
Example:
function handleClick() {
alert('Button clicked!');
}
function Button() {
return <button onClick={handleClick}>Click Me</button>;
}
Advanced React Concepts
React Hooks
React hooks were introduced in React 16.8 to enable functional components to manage state and side effects. Some essential hooks include:
- useState: Manages state in functional components.
- useEffect: Handles side effects like data fetching or subscriptions.
- useContext: Allows you to share values like themes or user data across the app without manually passing props.
Example of useState
and useEffect
:
import React, { useState, useEffect } from 'react';
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const timerId = setInterval(() => setSeconds((prev) => prev + 1), 1000);
return () => clearInterval(timerId); // Clean up on component unmount
}, []);
return <p>Time: {seconds} seconds</p>;
}
React Performance Optimization
Understanding Re-renders
React re-renders components when their state or props change. While this is necessary to keep the UI in sync with the data, excessive re-renders can lead to performance issues, especially in large applications.
React provides several optimization techniques:
- React.memo: A higher-order component that memoizes functional components, preventing unnecessary re-renders.
- useMemo and useCallback: These hooks help you memoize expensive calculations and functions, ensuring they’re only recalculated when necessary.
State Management in React
State management is crucial in React applications, especially as they grow in complexity. Managing state across multiple components can be challenging, but React provides several approaches to handle it:
- Local State: Each component manages its own state, typically with
useState
or class-basedsetState
. - Global State: For sharing state across components, you can use the Context API or third-party libraries like Redux.
React Testing and Debugging
Unit Testing with Jest and React Testing Library
React testing is an essential part of maintaining a high-quality application. Tools like Jest and React Testing Library help you test components, ensuring they behave as expected. React Testing Library focuses on testing components the way users interact with them.
Take the Ultimate React Quiz
It’s time to test your React knowledge with our ultimate React quiz! In the next section, we’ll walk you through a set of questions that cover everything from basic concepts to advanced topics.
Conclusion
Testing your React knowledge regularly is essential for growth as a developer. Whether you’re just starting or have years of experience, this quiz will help you identify areas where you excel and areas where you might need more practice. Keep learning and refining your React skills, and you’ll be able to build even more powerful and efficient applications.
0 Comments
No Comment Available