Online React Compiler with Tailwind CSS
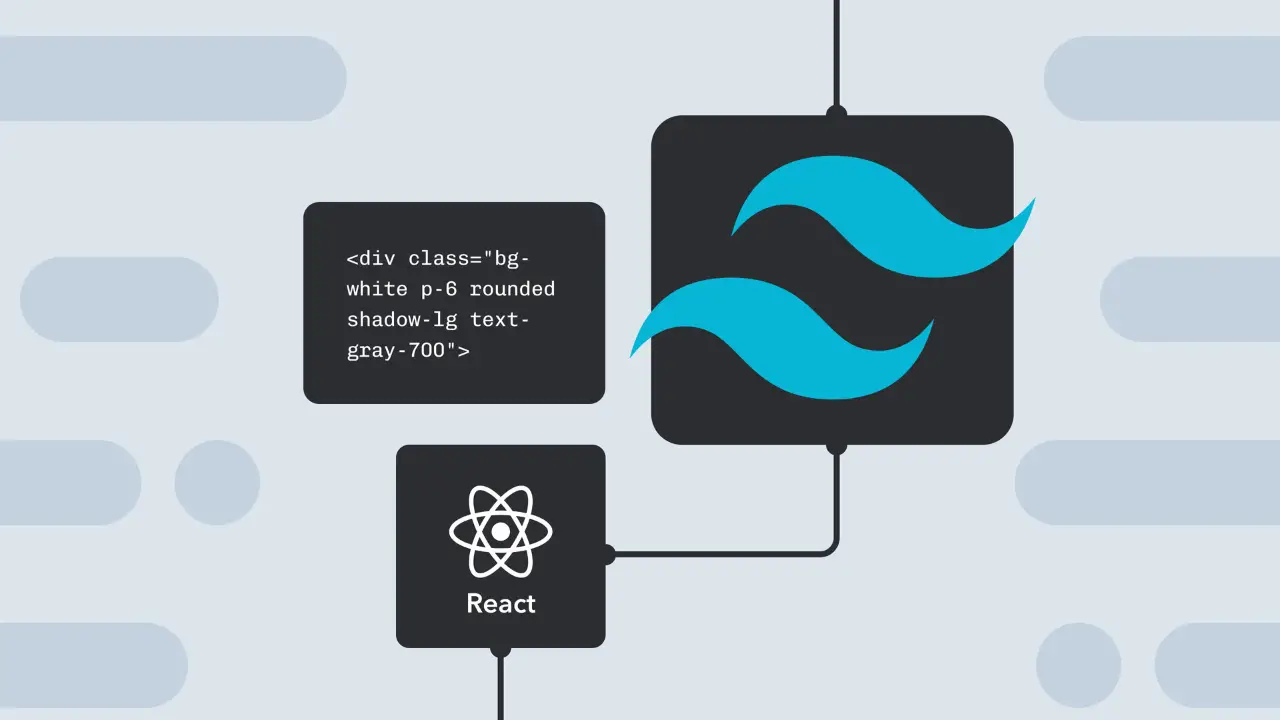
React and Tailwind CSS have revolutionized front-end development by enabling developers to create dynamic, responsive, and highly customizable user interfaces. An online React compiler with integrated Tailwind CSS takes this to the next level, providing an environment where you can write, compile, and preview your code in real-time.
In this blog post, we’ll explore the benefits of using an online React compiler with Tailwind CSS, how it works, and provide code examples to get you started. After completing this guide, you’ll understand how to use this tool for efficient development.
What Is an Online React Compiler with Tailwind CSS?
An online React compiler with Tailwind CSS is a web-based platform that lets you write and run React components styled with Tailwind CSS directly in your browser. It eliminates the need for local setup and provides a ready-made environment for:
- Experimenting with React and Tailwind CSS.
- Quickly test code snippets and prototypes.
- Share live previews with collaborators.
Popular online React compilers include CodeSandbox, StackBlitz, and custom-built tools for React and Tailwind CSS integration.
Benefits of Using an Online React Compiler with Tailwind CSS
1. No local setup required
You can avoid the hassle of installing Node.js, npm, or any other dependencies. Everything runs directly in your browser.
2. Real-time preview
Get instant feedback on your code. Changes are reflected in the output immediately, perfect for debugging and rapid prototyping.
3. Collaboration made easy
Shareable links let you collaborate with team members or showcase your work to clients seamlessly.
4. Integration with Tailwind CSS
Tailwind’s utility-first CSS framework can be implemented effortlessly, allowing for responsive and modern designs without writing custom CSS.
How to Use an Online React Compiler with Tailwind CSS
Here's a step-by-step guide to using an online React compiler with Tailwind CSS.
Step 1: Choose a compiler
Some of the best platforms for this purpose include:
- CodeSandbox: Offers built-in React and Tailwind CSS support.
- StackBlitz: Another great option for online React development.
- Play.js: Ideal for simple React projects.
Step 2: Set up Tailwind CSS
If Tailwind is not pre-installed on your compiler, you can add it manually:
1. Install Tailwind CSS using npm:
npm install tailwindcss postcss autoprefixer
2. Create a Tailwind CSS configuration file:
npx tailwindcss init
3. Add Tailwind to your CSS file:
@tailwind base;
@tailwind components;
@tailwind utilities;
Step 3: Write Your React Code
Now, you can start building React components styled with Tailwind CSS.
Example: Building a React Component with Tailwind CSS
Let’s create a simple Todo App using an online React compiler with Tailwind CSS.
Code Example:
import React, { useState } from 'react';
function TodoApp() {
const [todos, setTodos] = useState([]);
const [task, setTask] = useState('');
const addTodo = () => {
if (task) {
setTodos([...todos, task]);
setTask('');
}
};
return (
<div className="bg-gray-100 min-h-screen flex items-center justify-center">
<div className="bg-white p-6 rounded-lg shadow-lg w-full max-w-md">
<h1 className="text-2xl font-bold mb-4 text-center">Todo App</h1>
<div className="flex gap-2 mb-4">
<input
type="text"
value={task}
onChange={(e) => setTask(e.target.value)}
placeholder="Enter a task"
className="flex-grow border border-gray-300 p-2 rounded-lg"
/>
<button
onClick={addTodo}
className="bg-blue-500 text-white px-4 py-2 rounded-lg hover:bg-blue-600"
>
Add
</button>
</div>
<ul>
{todos.map((todo, index) => (
<li
key={index}
className="bg-gray-200 p-2 mb-2 rounded-lg flex items-center justify-between"
>
{todo}
</li>
))}
</ul>
</div>
</div>
);
}
export default TodoApp;
Explanation:
- Layout: The app is centered using Tailwind's utility classes such as
flex
,items-center
, andjustify-center
. - Styling: Tailwind's classes, such as
bg-gray-100
,rounded-lg
, andshadow-lg
, provide a modern look. - Functionality: The
addTodo
function updates the state with new tasks, anduseState
handles dynamic data.
Tips for using React and Tailwind CSS together
- Keep components modular Break large components into smaller pieces for better reusability and readability.
- Use Tailwind's responsive design Use Tailwind's responsive classes (e.g.,
md:
,lg:
) to adapt to different screen sizes. - Use Tailwind plugins Extend Tailwind's functionality with plugins like
@tailwindcss/forms
and@tailwindcss/typography
for advanced styling. - Shortcuts for common patterns Use Tailwind's shorthand classes to reduce code repetition. For example,
p-4
applies padding on all sides.
Conclusion
An online React compiler with Tailwind CSS is an invaluable tool for developers who want to quickly build, test, and share modern web applications. By combining the power of React’s dynamic components with Tailwind’s utility-first design, you can effortlessly create stunning and responsive interfaces.
Start experimenting with your favorite online compiler today and unlock the full potential of React and Tailwind CSS!
FAQ's
Can Tailwind and CSS be used together?
We use CSS variables extensively within Tailwind itself, so if you can use Tailwind, you can use native CSS variables. Learn more about the theme() function in our functions and directives documentation.
Is Tailwind still being used?
Both of them are being used for modern website styling. However, if you want to complete and deploy a project quickly, Bootstrap is suitable. But if you need advanced customization, a lot of CSS design flexibility, and complete control, Tailwind CSS is the best fit
Can we use Tailwind CSS in production?
For production, it's better to install Tailwind CSS and configure it to remove unused CSS, resulting in a much smaller, optimized CSS file.
Is Tailwind open source?
Tailwind CSS is an open-source CSS framework.
Tailwind CSS is an open-source CSS framework.
In this example, we're using the `flex` class to create a flex container, and the `w-1/2` class to set the width of each column to half of the container's width. We're also using different background colors to distinguish between the two columns.
0 Comments
No Comment Available